User-Provided Mesh Example
Here is an example script with a custom mesh provided as an array of coordinates. This should help you understand how meshes are defined in OpenAeroStruct and how to create them for your own custom planform shapes. This is an alternative to the helper-function approach described in Aerodynamic Optimization.
The following shows the portion of the example script in which the user provides the coordinates for the mesh. This example is for a wing with a kink and two distinct trapezoidal segments.
# -----------------------------------------------------------------------------
# CUSTOM MESH: Example mesh for a 2-segment wing with sweep
# -----------------------------------------------------------------------------
# Planform specifications
half_span = 12.0 # wing half-span in m
kink_location = 4.0 # spanwise location of the kink in m
root_chord = 6.0 # root chord in m
kink_chord = 3.0 # kink chord in m
tip_chord = 2.0 # tip chord in m
inboard_LE_sweep = 10.0 # inboard leading-edge sweep angle in deg
outboard_LE_sweep = -10.0 # outboard leading-edge sweep angle in deg
# Mesh specifications
nx = 5 # number of chordwise nodal points (should be odd)
ny_outboard = 9 # number of spanwise nodal points for the outboard segment
ny_inboard = 7 # number of spanwise nodal points for the inboard segment
# Initialize the 3-D mesh object. Indexing: Chordwise, spanwise, then the 3-D coordinates.
# We use ny_inboard+ny_outboard-1 because the 2 segments share the nodes where they connect.
mesh = np.zeros((nx, ny_inboard + ny_outboard - 1, 3))
# The form of this 3-D array can be confusing initially.
# For each node, we are providing the x, y, and z coordinates.
# x is streamwise, y is spanwise, and z is up.
# For example, the node for the leading edge at the tip would be specified as mesh[0, 0, :] = np.array([x, y, z]).
# And the node at the trailing edge at the root would be mesh[nx-1, ny-1, :] = np.array([x, y, z]).
# We only provide the right half of the wing here because we use symmetry.
# Print elements of the mesh to better understand the form.
####### THE Z-COORDINATES ######
# Assume no dihedral, so set the z-coordinate for all the points to 0.
mesh[:, :, 2] = 0.0
####### THE Y-COORDINATES ######
# Using uniform spacing for the spanwise locations of all the nodes within each of the two trapezoidal segments:
# Outboard
mesh[:, :ny_outboard, 1] = np.linspace(half_span, kink_location, ny_outboard)
# Inboard
mesh[:, ny_outboard : ny_outboard + ny_inboard, 1] = np.linspace(kink_location, 0, ny_inboard)[1:]
###### THE X-COORDINATES ######
# Start with the leading edge and create some intermediate arrays that we will use
x_LE = np.zeros(ny_inboard + ny_outboard - 1)
array_for_inboard_leading_edge_x_coord = np.linspace(0, kink_location, ny_inboard) * np.tan(
inboard_LE_sweep / 180.0 * np.pi
)
array_for_outboard_leading_edge_x_coord = (
np.linspace(0, half_span - kink_location, ny_outboard) * np.tan(outboard_LE_sweep / 180.0 * np.pi)
+ np.ones(ny_outboard) * array_for_inboard_leading_edge_x_coord[-1]
)
x_LE[:ny_inboard] = array_for_inboard_leading_edge_x_coord
x_LE[ny_inboard : ny_inboard + ny_outboard] = array_for_outboard_leading_edge_x_coord[1:]
# Then the trailing edge
x_TE = np.zeros(ny_inboard + ny_outboard - 1)
array_for_inboard_trailing_edge_x_coord = np.linspace(
array_for_inboard_leading_edge_x_coord[0] + root_chord,
array_for_inboard_leading_edge_x_coord[-1] + kink_chord,
ny_inboard,
)
array_for_outboard_trailing_edge_x_coord = np.linspace(
array_for_outboard_leading_edge_x_coord[0] + kink_chord,
array_for_outboard_leading_edge_x_coord[-1] + tip_chord,
ny_outboard,
)
x_TE[:ny_inboard] = array_for_inboard_trailing_edge_x_coord
x_TE[ny_inboard : ny_inboard + ny_outboard] = array_for_outboard_trailing_edge_x_coord[1:]
# # Quick plot to check leading and trailing edge x-coords
# plt.plot(x_LE, np.arange(0, ny_inboard+ny_outboard-1), marker='*')
# plt.plot(x_TE, np.arange(0, ny_inboard+ny_outboard-1), marker='*')
# plt.show()
# exit()
for i in range(0, ny_inboard + ny_outboard - 1):
mesh[:, i, 0] = np.linspace(np.flip(x_LE)[i], np.flip(x_TE)[i], nx)
# -----------------------------------------------------------------------------
# END MESH
# -----------------------------------------------------------------------------
The following shows a visualization of the mesh.
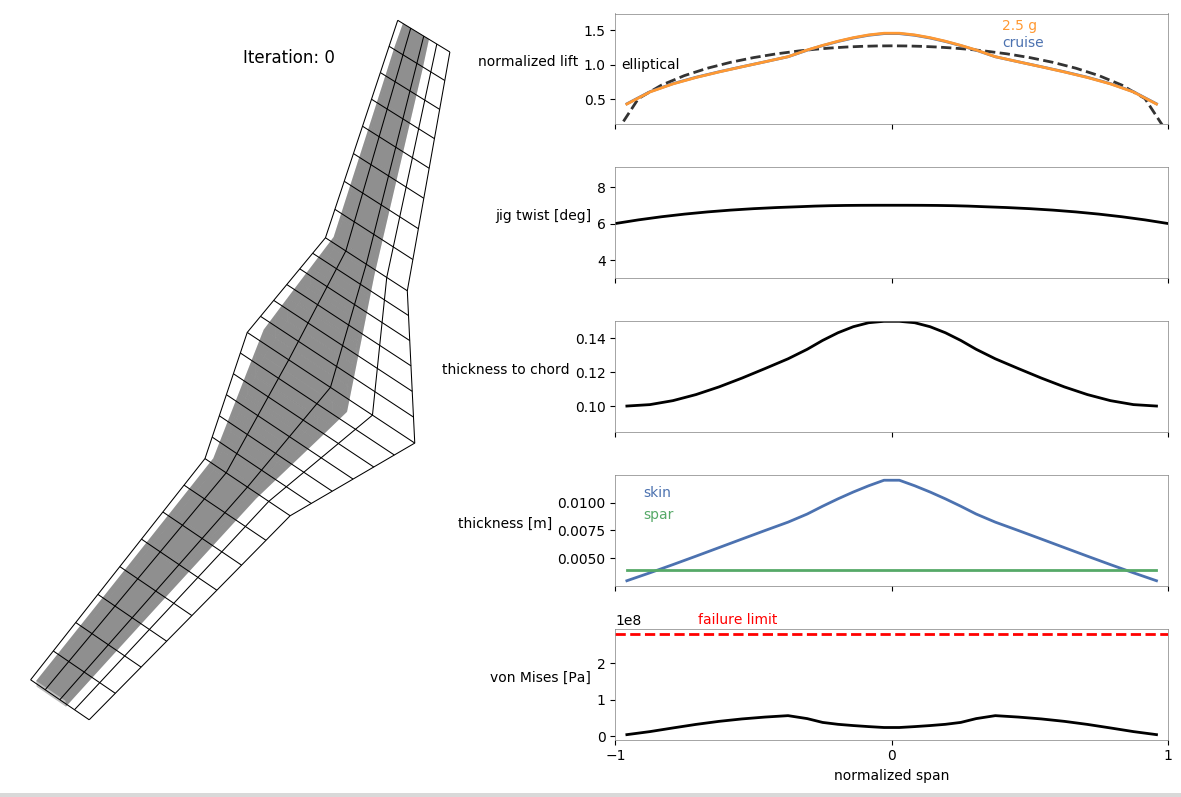
The complete script for the optimization is as follows. Make sure you go through the Aerostructural Optimization with Wingbox before trying to understand this setup.
import warnings
import matplotlib
warnings.filterwarnings('ignore')
matplotlib.use('Agg')
"""
This example script can be used to run a multipoint aerostructural (w/ wingbox) optimization for a custom user-provided mesh.
The fuel burn from the cruise flight-point is the objective function and a 2.5g
maneuver flight-point is used for the structural sizing.
After running the optimization, use the 'plot_wingbox.py' script in the utils/
directory (e.g., as 'python ../utils/plot_wingbox.py aerostruct.db' if running
from this directory) to visualize the results.
'plot_wingbox.py' is still under development and will probably not work as it is for other types of cases for now.
"""
import numpy as np
from openaerostruct.integration.aerostruct_groups import AerostructGeometry, AerostructPoint
from openaerostruct.structures.wingbox_fuel_vol_delta import WingboxFuelVolDelta
import openmdao.api as om
# docs checkpoint 0
# -----------------------------------------------------------------------------
# CUSTOM MESH: Example mesh for a 2-segment wing with sweep
# -----------------------------------------------------------------------------
# Planform specifications
half_span = 12.0 # wing half-span in m
kink_location = 4.0 # spanwise location of the kink in m
root_chord = 6.0 # root chord in m
kink_chord = 3.0 # kink chord in m
tip_chord = 2.0 # tip chord in m
inboard_LE_sweep = 10.0 # inboard leading-edge sweep angle in deg
outboard_LE_sweep = -10.0 # outboard leading-edge sweep angle in deg
# Mesh specifications
nx = 5 # number of chordwise nodal points (should be odd)
ny_outboard = 9 # number of spanwise nodal points for the outboard segment
ny_inboard = 7 # number of spanwise nodal points for the inboard segment
# Initialize the 3-D mesh object. Indexing: Chordwise, spanwise, then the 3-D coordinates.
# We use ny_inboard+ny_outboard-1 because the 2 segments share the nodes where they connect.
mesh = np.zeros((nx, ny_inboard + ny_outboard - 1, 3))
# The form of this 3-D array can be confusing initially.
# For each node, we are providing the x, y, and z coordinates.
# x is streamwise, y is spanwise, and z is up.
# For example, the node for the leading edge at the tip would be specified as mesh[0, 0, :] = np.array([x, y, z]).
# And the node at the trailing edge at the root would be mesh[nx-1, ny-1, :] = np.array([x, y, z]).
# We only provide the right half of the wing here because we use symmetry.
# Print elements of the mesh to better understand the form.
####### THE Z-COORDINATES ######
# Assume no dihedral, so set the z-coordinate for all the points to 0.
mesh[:, :, 2] = 0.0
####### THE Y-COORDINATES ######
# Using uniform spacing for the spanwise locations of all the nodes within each of the two trapezoidal segments:
# Outboard
mesh[:, :ny_outboard, 1] = np.linspace(half_span, kink_location, ny_outboard)
# Inboard
mesh[:, ny_outboard : ny_outboard + ny_inboard, 1] = np.linspace(kink_location, 0, ny_inboard)[1:]
###### THE X-COORDINATES ######
# Start with the leading edge and create some intermediate arrays that we will use
x_LE = np.zeros(ny_inboard + ny_outboard - 1)
array_for_inboard_leading_edge_x_coord = np.linspace(0, kink_location, ny_inboard) * np.tan(
inboard_LE_sweep / 180.0 * np.pi
)
array_for_outboard_leading_edge_x_coord = (
np.linspace(0, half_span - kink_location, ny_outboard) * np.tan(outboard_LE_sweep / 180.0 * np.pi)
+ np.ones(ny_outboard) * array_for_inboard_leading_edge_x_coord[-1]
)
x_LE[:ny_inboard] = array_for_inboard_leading_edge_x_coord
x_LE[ny_inboard : ny_inboard + ny_outboard] = array_for_outboard_leading_edge_x_coord[1:]
# Then the trailing edge
x_TE = np.zeros(ny_inboard + ny_outboard - 1)
array_for_inboard_trailing_edge_x_coord = np.linspace(
array_for_inboard_leading_edge_x_coord[0] + root_chord,
array_for_inboard_leading_edge_x_coord[-1] + kink_chord,
ny_inboard,
)
array_for_outboard_trailing_edge_x_coord = np.linspace(
array_for_outboard_leading_edge_x_coord[0] + kink_chord,
array_for_outboard_leading_edge_x_coord[-1] + tip_chord,
ny_outboard,
)
x_TE[:ny_inboard] = array_for_inboard_trailing_edge_x_coord
x_TE[ny_inboard : ny_inboard + ny_outboard] = array_for_outboard_trailing_edge_x_coord[1:]
# # Quick plot to check leading and trailing edge x-coords
# plt.plot(x_LE, np.arange(0, ny_inboard+ny_outboard-1), marker='*')
# plt.plot(x_TE, np.arange(0, ny_inboard+ny_outboard-1), marker='*')
# plt.show()
# exit()
for i in range(0, ny_inboard + ny_outboard - 1):
mesh[:, i, 0] = np.linspace(np.flip(x_LE)[i], np.flip(x_TE)[i], nx)
# -----------------------------------------------------------------------------
# END MESH
# -----------------------------------------------------------------------------
# docs checkpoint 1
# -----------------------------------------------------------------------------
# On to the problem setup (this is the same setup used for the Q400 example)
# -----------------------------------------------------------------------------
# Provide coordinates for a portion of an airfoil for the wingbox cross-section as an nparray with dtype=complex (to work with the complex-step approximation for derivatives).
# These should be for an airfoil with the chord scaled to 1.
# We use the 10% to 60% portion of the NASA SC2-0612 airfoil for this case
# We use the coordinates available from airfoiltools.com. Using such a large number of coordinates is not necessary.
# The first and last x-coordinates of the upper and lower surfaces must be the same
# fmt: off
upper_x = np.array([0.1, 0.11, 0.12, 0.13, 0.14, 0.15, 0.16, 0.17, 0.18, 0.19, 0.2, 0.21, 0.22, 0.23, 0.24, 0.25, 0.26, 0.27, 0.28, 0.29, 0.3, 0.31, 0.32, 0.33, 0.34, 0.35, 0.36, 0.37, 0.38, 0.39, 0.4, 0.41, 0.42, 0.43, 0.44, 0.45, 0.46, 0.47, 0.48, 0.49, 0.5, 0.51, 0.52, 0.53, 0.54, 0.55, 0.56, 0.57, 0.58, 0.59, 0.6], dtype="complex128")
lower_x = np.array([0.1, 0.11, 0.12, 0.13, 0.14, 0.15, 0.16, 0.17, 0.18, 0.19, 0.2, 0.21, 0.22, 0.23, 0.24, 0.25, 0.26, 0.27, 0.28, 0.29, 0.3, 0.31, 0.32, 0.33, 0.34, 0.35, 0.36, 0.37, 0.38, 0.39, 0.4, 0.41, 0.42, 0.43, 0.44, 0.45, 0.46, 0.47, 0.48, 0.49, 0.5, 0.51, 0.52, 0.53, 0.54, 0.55, 0.56, 0.57, 0.58, 0.59, 0.6], dtype="complex128")
upper_y = np.array([ 0.0447, 0.046, 0.0472, 0.0484, 0.0495, 0.0505, 0.0514, 0.0523, 0.0531, 0.0538, 0.0545, 0.0551, 0.0557, 0.0563, 0.0568, 0.0573, 0.0577, 0.0581, 0.0585, 0.0588, 0.0591, 0.0593, 0.0595, 0.0597, 0.0599, 0.06, 0.0601, 0.0602, 0.0602, 0.0602, 0.0602, 0.0602, 0.0601, 0.06, 0.0599, 0.0598, 0.0596, 0.0594, 0.0592, 0.0589, 0.0586, 0.0583, 0.058, 0.0576, 0.0572, 0.0568, 0.0563, 0.0558, 0.0553, 0.0547, 0.0541], dtype="complex128") # noqa: E201, E241
lower_y = np.array([-0.0447, -0.046, -0.0473, -0.0485, -0.0496, -0.0506, -0.0515, -0.0524, -0.0532, -0.054, -0.0547, -0.0554, -0.056, -0.0565, -0.057, -0.0575, -0.0579, -0.0583, -0.0586, -0.0589, -0.0592, -0.0594, -0.0595, -0.0596, -0.0597, -0.0598, -0.0598, -0.0598, -0.0598, -0.0597, -0.0596, -0.0594, -0.0592, -0.0589, -0.0586, -0.0582, -0.0578, -0.0573, -0.0567, -0.0561, -0.0554, -0.0546, -0.0538, -0.0529, -0.0519, -0.0509, -0.0497, -0.0485, -0.0472, -0.0458, -0.0444], dtype="complex128")
# fmt: on
surf_dict = {
# Wing definition
"name": "wing", # name of the surface
"symmetry": True, # if true, model one half of wing
"S_ref_type": "wetted", # how we compute the wing area,
# can be 'wetted' or 'projected'
"mesh": mesh,
"twist_cp": np.array([6.0, 7.0, 7.0, 7.0]),
"fem_model_type": "wingbox",
"data_x_upper": upper_x,
"data_x_lower": lower_x,
"data_y_upper": upper_y,
"data_y_lower": lower_y,
"spar_thickness_cp": np.array([0.004, 0.004, 0.004, 0.004]), # [m]
"skin_thickness_cp": np.array([0.003, 0.006, 0.010, 0.012]), # [m]
"original_wingbox_airfoil_t_over_c": 0.12,
# Aerodynamic deltas.
# These CL0 and CD0 values are added to the CL and CD
# obtained from aerodynamic analysis of the surface to get
# the total CL and CD.
# These CL0 and CD0 values do not vary wrt alpha.
# They can be used to account for things that are not included, such as contributions from the fuselage, nacelles, tail surfaces, etc.
"CL0": 0.0,
"CD0": 0.0142,
"with_viscous": True, # if true, compute viscous drag
"with_wave": True, # if true, compute wave drag
# Airfoil properties for viscous drag calculation
"k_lam": 0.05, # percentage of chord with laminar
# flow, used for viscous drag
"c_max_t": 0.38, # chordwise location of maximum thickness
"t_over_c_cp": np.array([0.1, 0.1, 0.15, 0.15]),
# Structural values are based on aluminum 7075
"E": 73.1e9, # [Pa] Young's modulus
"G": (73.1e9 / 2 / 1.33), # [Pa] shear modulus (calculated using E and the Poisson's ratio here)
"yield": (420.0e6 / 1.5), # [Pa] allowable yield stress
"mrho": 2.78e3, # [kg/m^3] material density
"strength_factor_for_upper_skin": 1.0, # the yield stress is multiplied by this factor for the upper skin
"wing_weight_ratio": 1.25,
"exact_failure_constraint": False, # if false, use KS function
"struct_weight_relief": True,
"distributed_fuel_weight": True,
"fuel_density": 803.0, # [kg/m^3] fuel density (only needed if the fuel-in-wing volume constraint is used)
"Wf_reserve": 500.0, # [kg] reserve fuel mass
}
surfaces = [surf_dict]
# Create the problem and assign the model group
prob = om.Problem()
# Add problem information as an independent variables component
indep_var_comp = om.IndepVarComp()
indep_var_comp.add_output("v", val=np.array([0.5 * 310.95, 0.3 * 340.294]), units="m/s")
indep_var_comp.add_output("alpha", val=0.0, units="deg")
indep_var_comp.add_output("alpha_maneuver", val=0.0, units="deg")
indep_var_comp.add_output("Mach_number", val=np.array([0.5, 0.3]))
indep_var_comp.add_output(
"re",
val=np.array([0.569 * 310.95 * 0.5 * 1.0 / (1.56 * 1e-5), 1.225 * 340.294 * 0.3 * 1.0 / (1.81206 * 1e-5)]),
units="1/m",
)
indep_var_comp.add_output("rho", val=np.array([0.569, 1.225]), units="kg/m**3")
indep_var_comp.add_output("CT", val=0.43 / 3600, units="1/s")
indep_var_comp.add_output("R", val=2e6, units="m")
indep_var_comp.add_output("W0", val=25400 + surf_dict["Wf_reserve"], units="kg")
indep_var_comp.add_output("speed_of_sound", val=np.array([310.95, 340.294]), units="m/s")
indep_var_comp.add_output("load_factor", val=np.array([1.0, 2.5]))
indep_var_comp.add_output("empty_cg", val=np.zeros((3)), units="m")
indep_var_comp.add_output("fuel_mass", val=3000.0, units="kg")
prob.model.add_subsystem("prob_vars", indep_var_comp, promotes=["*"])
# Loop over each surface in the surfaces list
for surface in surfaces:
# Get the surface name and create a group to contain components
# only for this surface
name = surface["name"]
aerostruct_group = AerostructGeometry(surface=surface)
# Add group to the problem with the name of the surface.
prob.model.add_subsystem(name, aerostruct_group)
# Loop through and add a certain number of aerostruct points
for i in range(2):
point_name = "AS_point_{}".format(i)
# Connect the parameters within the model for each aerostruct point
# Create the aero point group and add it to the model
AS_point = AerostructPoint(surfaces=surfaces, internally_connect_fuelburn=False)
prob.model.add_subsystem(point_name, AS_point)
# Connect flow properties to the analysis point
prob.model.connect("v", point_name + ".v", src_indices=[i])
prob.model.connect("Mach_number", point_name + ".Mach_number", src_indices=[i])
prob.model.connect("re", point_name + ".re", src_indices=[i])
prob.model.connect("rho", point_name + ".rho", src_indices=[i])
prob.model.connect("CT", point_name + ".CT")
prob.model.connect("R", point_name + ".R")
prob.model.connect("W0", point_name + ".W0")
prob.model.connect("speed_of_sound", point_name + ".speed_of_sound", src_indices=[i])
prob.model.connect("empty_cg", point_name + ".empty_cg")
prob.model.connect("load_factor", point_name + ".load_factor", src_indices=[i])
prob.model.connect("fuel_mass", point_name + ".total_perf.L_equals_W.fuelburn")
prob.model.connect("fuel_mass", point_name + ".total_perf.CG.fuelburn")
for surface in surfaces:
name = surface["name"]
if surf_dict["distributed_fuel_weight"]:
prob.model.connect("load_factor", point_name + ".coupled.load_factor", src_indices=[i])
com_name = point_name + "." + name + "_perf."
prob.model.connect(
name + ".local_stiff_transformed", point_name + ".coupled." + name + ".local_stiff_transformed"
)
prob.model.connect(name + ".nodes", point_name + ".coupled." + name + ".nodes")
# Connect aerodynamic mesh to coupled group mesh
prob.model.connect(name + ".mesh", point_name + ".coupled." + name + ".mesh")
if surf_dict["struct_weight_relief"]:
prob.model.connect(name + ".element_mass", point_name + ".coupled." + name + ".element_mass")
# Connect performance calculation variables
prob.model.connect(name + ".nodes", com_name + "nodes")
prob.model.connect(name + ".cg_location", point_name + "." + "total_perf." + name + "_cg_location")
prob.model.connect(name + ".structural_mass", point_name + "." + "total_perf." + name + "_structural_mass")
# Connect wingbox properties to von Mises stress calcs
prob.model.connect(name + ".Qz", com_name + "Qz")
prob.model.connect(name + ".J", com_name + "J")
prob.model.connect(name + ".A_enc", com_name + "A_enc")
prob.model.connect(name + ".htop", com_name + "htop")
prob.model.connect(name + ".hbottom", com_name + "hbottom")
prob.model.connect(name + ".hfront", com_name + "hfront")
prob.model.connect(name + ".hrear", com_name + "hrear")
prob.model.connect(name + ".spar_thickness", com_name + "spar_thickness")
prob.model.connect(name + ".t_over_c", com_name + "t_over_c")
prob.model.connect("alpha", "AS_point_0" + ".alpha")
prob.model.connect("alpha_maneuver", "AS_point_1" + ".alpha")
# Here we add the fuel volume constraint componenet to the model
prob.model.add_subsystem("fuel_vol_delta", WingboxFuelVolDelta(surface=surface))
prob.model.connect("wing.struct_setup.fuel_vols", "fuel_vol_delta.fuel_vols")
prob.model.connect("AS_point_0.fuelburn", "fuel_vol_delta.fuelburn")
if surf_dict["distributed_fuel_weight"]:
prob.model.connect("wing.struct_setup.fuel_vols", "AS_point_0.coupled.wing.struct_states.fuel_vols")
prob.model.connect("fuel_mass", "AS_point_0.coupled.wing.struct_states.fuel_mass")
prob.model.connect("wing.struct_setup.fuel_vols", "AS_point_1.coupled.wing.struct_states.fuel_vols")
prob.model.connect("fuel_mass", "AS_point_1.coupled.wing.struct_states.fuel_mass")
comp = om.ExecComp("fuel_diff = (fuel_mass - fuelburn) / fuelburn", units="kg")
prob.model.add_subsystem("fuel_diff", comp, promotes_inputs=["fuel_mass"], promotes_outputs=["fuel_diff"])
prob.model.connect("AS_point_0.fuelburn", "fuel_diff.fuelburn")
## Use these settings if you do not have pyOptSparse or SNOPT
prob.driver = om.ScipyOptimizeDriver()
prob.driver.options["optimizer"] = "SLSQP"
prob.driver.options["tol"] = 1e-4
recorder = om.SqliteRecorder("aerostruct.db")
prob.driver.add_recorder(recorder)
# We could also just use prob.driver.recording_options['includes']=['*'] here, but for large meshes the database file becomes extremely large. So we just select the variables we need.
prob.driver.recording_options["includes"] = [
"alpha",
"rho",
"v",
"cg",
"AS_point_1.cg",
"AS_point_0.cg",
"AS_point_0.coupled.wing_loads.loads",
"AS_point_1.coupled.wing_loads.loads",
"AS_point_0.coupled.wing.normals",
"AS_point_1.coupled.wing.normals",
"AS_point_0.coupled.wing.widths",
"AS_point_1.coupled.wing.widths",
"AS_point_0.coupled.aero_states.wing_sec_forces",
"AS_point_1.coupled.aero_states.wing_sec_forces",
"AS_point_0.wing_perf.CL1",
"AS_point_1.wing_perf.CL1",
"AS_point_0.coupled.wing.S_ref",
"AS_point_1.coupled.wing.S_ref",
"wing.geometry.twist",
"wing.mesh",
"wing.skin_thickness",
"wing.spar_thickness",
"wing.t_over_c",
"wing.structural_mass",
"AS_point_0.wing_perf.vonmises",
"AS_point_1.wing_perf.vonmises",
"AS_point_0.coupled.wing.def_mesh",
"AS_point_1.coupled.wing.def_mesh",
]
prob.driver.recording_options["record_objectives"] = True
prob.driver.recording_options["record_constraints"] = True
prob.driver.recording_options["record_desvars"] = True
prob.driver.recording_options["record_inputs"] = True
prob.model.add_objective("AS_point_0.fuelburn", scaler=1e-5)
prob.model.add_design_var("wing.twist_cp", lower=-15.0, upper=15.0, scaler=0.1)
prob.model.add_design_var("wing.spar_thickness_cp", lower=0.003, upper=0.1, scaler=1e2)
prob.model.add_design_var("wing.skin_thickness_cp", lower=0.003, upper=0.1, scaler=1e2)
prob.model.add_design_var("wing.geometry.t_over_c_cp", lower=0.07, upper=0.2, scaler=10.0)
prob.model.add_design_var("fuel_mass", lower=0.0, upper=2e5, scaler=1e-5)
prob.model.add_design_var("alpha_maneuver", lower=-15.0, upper=15)
prob.model.add_constraint("AS_point_0.CL", equals=0.6)
prob.model.add_constraint("AS_point_1.L_equals_W", equals=0.0)
prob.model.add_constraint("AS_point_1.wing_perf.failure", upper=0.0)
prob.model.add_constraint("fuel_vol_delta.fuel_vol_delta", lower=0.0)
prob.model.add_constraint("fuel_diff", equals=0.0)
# Set up the problem
prob.setup()
/home/docs/checkouts/readthedocs.org/user_builds/mdolab-openaerostruct/envs/latest/lib/python3.11/site-packages/openmdao/core/system.py:2422: PromotionWarning:'AS_point_0.coupled.wing.struct_states' <class SpatialBeamStates>: input variable 'load_factor', promoted using 'load_factor', was already promoted using 'load_factor'. /home/docs/checkouts/readthedocs.org/user_builds/mdolab-openaerostruct/envs/latest/lib/python3.11/site-packages/openmdao/core/system.py:2422: PromotionWarning:'AS_point_0.coupled.wing.struct_states' <class SpatialBeamStates>: input variable 'nodes', promoted using 'nodes', was already promoted using 'nodes'. /home/docs/checkouts/readthedocs.org/user_builds/mdolab-openaerostruct/envs/latest/lib/python3.11/site-packages/openmdao/core/system.py:2422: PromotionWarning:'AS_point_1.coupled.wing.struct_states' <class SpatialBeamStates>: input variable 'load_factor', promoted using 'load_factor', was already promoted using 'load_factor'. /home/docs/checkouts/readthedocs.org/user_builds/mdolab-openaerostruct/envs/latest/lib/python3.11/site-packages/openmdao/core/system.py:2422: PromotionWarning:'AS_point_1.coupled.wing.struct_states' <class SpatialBeamStates>: input variable 'nodes', promoted using 'nodes', was already promoted using 'nodes'. /home/docs/checkouts/readthedocs.org/user_builds/mdolab-openaerostruct/envs/latest/lib/python3.11/site-packages/openmdao/core/driver.py:743: OpenMDAOWarning:ScipyOptimizeDriver: No matches for pattern 'cg' in recording_options['includes'].
# change linear solver for aerostructural coupled adjoint
prob.model.AS_point_0.coupled.linear_solver = om.LinearBlockGS(iprint=0, maxiter=30, use_aitken=True)
prob.model.AS_point_1.coupled.linear_solver = om.LinearBlockGS(iprint=0, maxiter=30, use_aitken=True)
# om.view_model(prob)
# prob.check_partials(form='central', compact_print=True)
prob.run_driver()
================== AS_point_0.coupled ================== NL: NLBGS 1 ; 59819.4728 1 NL: NLBGS 2 ; 59187.3066 0.9894321 NL: NLBGS 3 ; 1879.7489 0.0314236956 NL: NLBGS 4 ; 47.0388256 0.000786346375 NL: NLBGS 5 ; 0.117150412 1.95839927e-06 NL: NLBGS 6 ; 0.00466493664 7.798358e-08 NL: NLBGS 7 ; 3.90004533e-05 6.51969192e-10 NL: NLBGS 8 ; 4.64470169e-08 7.7645313e-13 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 63961.0672 1 NL: NLBGS 2 ; 54760.1129 0.85614758 NL: NLBGS 3 ; 1601.08786 0.0250322256 NL: NLBGS 4 ; 37.0946936 0.00057995739 NL: NLBGS 5 ; 0.0861602861 1.34707393e-06 NL: NLBGS 6 ; 0.00311660643 4.8726617e-08 NL: NLBGS 7 ; 2.72242833e-05 4.25638353e-10 NL: NLBGS 8 ; 2.65633562e-08 4.15305082e-13 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 1.27431151e-09 1 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 7.73898743e-10 1 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 13182.9265 1 NL: NLBGS 2 ; 12203.3035 0.925690025 NL: NLBGS 3 ; 1931.43789 0.146510556 NL: NLBGS 4 ; 81.7125596 0.0061983627 NL: NLBGS 5 ; 0.938797863 7.12131609e-05 NL: NLBGS 6 ; 0.0805607708 6.11099296e-06 NL: NLBGS 7 ; 0.00202640221 1.5371414e-07 NL: NLBGS 8 ; 2.40681435e-05 1.82570567e-09 NL: NLBGS 9 ; 1.55115374e-06 1.1766384e-10 NL: NLBGS 10 ; 1.21665613e-07 9.22902919e-12 NL: NLBGS 11 ; 4.41000751e-09 3.34524169e-13 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 100627.776 1 NL: NLBGS 2 ; 98695.2584 0.980795387 NL: NLBGS 3 ; 16329.0591 0.162271887 NL: NLBGS 4 ; 876.928461 0.0087145766 NL: NLBGS 5 ; 32.6985144 0.000324945216 NL: NLBGS 6 ; 1.59196514 1.58203352e-05 NL: NLBGS 7 ; 0.0535616814 5.32275318e-07 NL: NLBGS 8 ; 0.00288506466 2.86706592e-08 NL: NLBGS 9 ; 4.41671969e-05 4.38916557e-10 NL: NLBGS 10 ; 9.39914238e-06 9.34050494e-11 NL: NLBGS 11 ; 1.61676089e-06 1.60667457e-11 NL: NLBGS 12 ; 3.87733381e-08 3.8531447e-13 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 4455.4879 1 NL: NLBGS 2 ; 4346.71715 0.975587242 NL: NLBGS 3 ; 496.589363 0.111455664 NL: NLBGS 4 ; 16.0643834 0.00360552733 NL: NLBGS 5 ; 0.127301542 2.85718522e-05 NL: NLBGS 6 ; 0.0094779491 2.1272528e-06 NL: NLBGS 7 ; 0.000111216458 2.4961679e-08 NL: NLBGS 8 ; 6.99317961e-07 1.56956539e-10 NL: NLBGS 9 ; 5.52767667e-08 1.24064453e-11 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 12363.017 1 NL: NLBGS 2 ; 11539.1768 0.933362523 NL: NLBGS 3 ; 1673.26519 0.135344405 NL: NLBGS 4 ; 45.9036559 0.00371298169 NL: NLBGS 5 ; 0.266990112 2.15958702e-05 NL: NLBGS 6 ; 0.0298712644 2.41617918e-06 NL: NLBGS 7 ; 0.000491091982 3.97226648e-08 NL: NLBGS 8 ; 7.73339501e-06 6.25526519e-10 NL: NLBGS 9 ; 4.25276941e-07 3.43991228e-11 NL: NLBGS 10 ; 1.32346513e-08 1.07050337e-12 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 7428.56475 1 NL: NLBGS 2 ; 7454.23582 1.00345573 NL: NLBGS 3 ; 561.08328 0.0755305095 NL: NLBGS 4 ; 41.8942523 0.00563961595 NL: NLBGS 5 ; 0.153419528 2.06526474e-05 NL: NLBGS 6 ; 0.00375465043 5.05434167e-07 NL: NLBGS 7 ; 0.000229436244 3.08856761e-08 NL: NLBGS 8 ; 6.63641617e-07 8.93364519e-11 NL: NLBGS 9 ; 1.19693845e-08 1.61126475e-12 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 7417.14914 1 NL: NLBGS 2 ; 6934.96737 0.934990957 NL: NLBGS 3 ; 797.988861 0.107587005 NL: NLBGS 4 ; 45.6094678 0.00614919115 NL: NLBGS 5 ; 0.363661014 4.90297562e-05 NL: NLBGS 6 ; 0.0135853845 1.83161808e-06 NL: NLBGS 7 ; 0.000577758607 7.78949697e-08 NL: NLBGS 8 ; 3.2215825e-06 4.34342419e-10 NL: NLBGS 9 ; 1.2331219e-07 1.66252811e-11 NL: NLBGS 10 ; 7.50454455e-09 1.01178288e-12 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 6235.14415 1 NL: NLBGS 2 ; 6252.50818 1.00278486 NL: NLBGS 3 ; 431.866279 0.0692632388 NL: NLBGS 4 ; 34.2799289 0.00549785668 NL: NLBGS 5 ; 0.131774704 2.11341872e-05 NL: NLBGS 6 ; 0.00202154181 3.24217333e-07 NL: NLBGS 7 ; 0.000139764864 2.24156588e-08 NL: NLBGS 8 ; 3.3480034e-07 5.36956855e-11 NL: NLBGS 9 ; 4.9678542e-09 7.96750496e-13 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 6251.91828 1 NL: NLBGS 2 ; 5823.80919 0.931523563 NL: NLBGS 3 ; 458.363403 0.0733156421 NL: NLBGS 4 ; 41.1887122 0.00658817188 NL: NLBGS 5 ; 0.258966219 4.14218817e-05 NL: NLBGS 6 ; 0.00167739156 2.68300302e-07 NL: NLBGS 7 ; 0.000125295775 2.00411729e-08 NL: NLBGS 8 ; 2.59673786e-07 4.15350576e-11 NL: NLBGS 9 ; 1.97211551e-09 3.15441664e-13 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 1678.44974 1 NL: NLBGS 2 ; 1658.03274 0.987835798 NL: NLBGS 3 ; 116.719706 0.0695401852 NL: NLBGS 4 ; 9.16697922 0.00546157504 NL: NLBGS 5 ; 0.0377886482 2.25140183e-05 NL: NLBGS 6 ; 0.000617224893 3.67735105e-07 NL: NLBGS 7 ; 4.201249e-05 2.5030532e-08 NL: NLBGS 8 ; 9.08534933e-08 5.41294095e-11 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 1831.45166 1 NL: NLBGS 2 ; 1583.994 0.864884413 NL: NLBGS 3 ; 112.379987 0.0613611537 NL: NLBGS 4 ; 10.5961848 0.00578567539 NL: NLBGS 5 ; 0.0718780393 3.92464845e-05 NL: NLBGS 6 ; 0.000717753499 3.91904146e-07 NL: NLBGS 7 ; 5.491392e-05 2.99838216e-08 NL: NLBGS 8 ; 4.89494171e-08 2.67271139e-11 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 177.930154 1 NL: NLBGS 2 ; 178.434393 1.00283391 NL: NLBGS 3 ; 11.4530251 0.0643680952 NL: NLBGS 4 ; 0.946669241 0.00532045423 NL: NLBGS 5 ; 0.00351967743 1.97812307e-05 NL: NLBGS 6 ; 3.35837686e-05 1.8874692e-07 NL: NLBGS 7 ; 2.51116352e-06 1.41131982e-08 NL: NLBGS 8 ; 5.46876943e-09 3.07354841e-11 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 177.908069 1 NL: NLBGS 2 ; 163.75034 0.920421095 NL: NLBGS 3 ; 12.9869972 0.0729983595 NL: NLBGS 4 ; 1.24994707 0.00702580316 NL: NLBGS 5 ; 0.00743786787 4.18073666e-05 NL: NLBGS 6 ; 9.6816413e-05 5.4419349e-07 NL: NLBGS 7 ; 7.66561369e-06 4.30874987e-08 NL: NLBGS 8 ; 1.40097173e-08 7.87469469e-11 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 1019.56826 1 NL: NLBGS 2 ; 1024.03283 1.00437888 NL: NLBGS 3 ; 68.3257349 0.0670143802 NL: NLBGS 4 ; 5.51147411 0.00540569409 NL: NLBGS 5 ; 0.0213857463 2.09752963e-05 NL: NLBGS 6 ; 0.000256983853 2.52051641e-07 NL: NLBGS 7 ; 1.84332724e-05 1.80794883e-08 NL: NLBGS 8 ; 4.09302621e-08 4.01447002e-11 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 1015.33539 1 NL: NLBGS 2 ; 951.447887 0.93707744 NL: NLBGS 3 ; 71.9974815 0.0709100483 NL: NLBGS 4 ; 6.86553257 0.00676183716 NL: NLBGS 5 ; 0.0439640206 4.32999983e-05 NL: NLBGS 6 ; 0.000522836787 5.14939982e-07 NL: NLBGS 7 ; 4.08338884e-05 4.02171428e-08 NL: NLBGS 8 ; 6.54140493e-08 6.44260508e-11 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 4551.01002 1 NL: NLBGS 2 ; 4576.00784 1.00549281 NL: NLBGS 3 ; 306.11985 0.0672641566 NL: NLBGS 4 ; 24.2794342 0.00533495512 NL: NLBGS 5 ; 0.0928297501 2.0397615e-05 NL: NLBGS 6 ; 0.00109083731 2.39691257e-07 NL: NLBGS 7 ; 7.69361035e-05 1.69052811e-08 NL: NLBGS 8 ; 1.83501525e-07 4.0321055e-11 NL: NLBGS 9 ; 2.05485995e-09 4.51517343e-13 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 4503.59894 1 NL: NLBGS 2 ; 4234.91448 0.940340056 NL: NLBGS 3 ; 326.67644 0.0725367521 NL: NLBGS 4 ; 30.4250772 0.00675572529 NL: NLBGS 5 ; 0.202973049 4.50690774e-05 NL: NLBGS 6 ; 0.00203719361 4.52347919e-07 NL: NLBGS 7 ; 0.000155687463 3.45695665e-08 NL: NLBGS 8 ; 2.92297744e-07 6.49031471e-11 NL: NLBGS 9 ; 1.74593807e-09 3.87676188e-13 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 3359.11605 1 NL: NLBGS 2 ; 3377.85822 1.00557949 NL: NLBGS 3 ; 225.013885 0.066986041 NL: NLBGS 4 ; 18.0166534 0.00536351027 NL: NLBGS 5 ; 0.0658387616 1.96000259e-05 NL: NLBGS 6 ; 0.000837425599 2.49299395e-07 NL: NLBGS 7 ; 5.9477678e-05 1.77063481e-08 NL: NLBGS 8 ; 1.45142203e-07 4.32084516e-11 NL: NLBGS 9 ; 1.78190163e-09 5.3046742e-13 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 3323.72812 1 NL: NLBGS 2 ; 3126.0042 0.940511405 NL: NLBGS 3 ; 239.791305 0.0721452827 NL: NLBGS 4 ; 22.4651391 0.00675901829 NL: NLBGS 5 ; 0.138156923 4.15668545e-05 NL: NLBGS 6 ; 0.0014815623 4.45753157e-07 NL: NLBGS 7 ; 0.000113000827 3.3998216e-08 NL: NLBGS 8 ; 1.48328177e-07 4.46270488e-11 NL: NLBGS 9 ; 1.53465784e-09 4.61727852e-13 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 2601.22546 1 NL: NLBGS 2 ; 2617.1098 1.00610648 NL: NLBGS 3 ; 173.279214 0.0666144541 NL: NLBGS 4 ; 13.7046073 0.00526851961 NL: NLBGS 5 ; 0.0501319066 1.92724189e-05 NL: NLBGS 6 ; 0.000603949289 2.32178755e-07 NL: NLBGS 7 ; 4.25489211e-05 1.635726e-08 NL: NLBGS 8 ; 1.01842791e-07 3.91518508e-11 NL: NLBGS 9 ; 1.14522167e-09 4.40262364e-13 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 2550.86864 1 NL: NLBGS 2 ; 2401.74168 0.941538752 NL: NLBGS 3 ; 189.445917 0.0742672179 NL: NLBGS 4 ; 17.6187306 0.00690695332 NL: NLBGS 5 ; 0.111872837 4.38567613e-05 NL: NLBGS 6 ; 0.00115028288 4.50937716e-07 NL: NLBGS 7 ; 8.78614957e-05 3.44437554e-08 NL: NLBGS 8 ; 1.68576492e-07 6.60859165e-11 NL: NLBGS 9 ; 1.10019461e-09 4.31301945e-13 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 1681.10361 1 NL: NLBGS 2 ; 1691.57222 1.00622722 NL: NLBGS 3 ; 111.958257 0.0665980708 NL: NLBGS 4 ; 8.92973623 0.00531182979 NL: NLBGS 5 ; 0.0320292429 1.90525098e-05 NL: NLBGS 6 ; 0.000398077407 2.36795284e-07 NL: NLBGS 7 ; 2.82479523e-05 1.68032191e-08 NL: NLBGS 8 ; 6.91927109e-08 4.11590996e-11 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 1647.82691 1 NL: NLBGS 2 ; 1552.03497 0.941867715 NL: NLBGS 3 ; 122.353976 0.074251716 NL: NLBGS 4 ; 11.4468683 0.00694664482 NL: NLBGS 5 ; 0.0697258954 4.23138467e-05 NL: NLBGS 6 ; 0.0007521354 4.56440779e-07 NL: NLBGS 7 ; 5.75020147e-05 3.48956642e-08 NL: NLBGS 8 ; 9.47556755e-08 5.75034154e-11 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 534.807124 1 NL: NLBGS 2 ; 536.319268 1.00282746 NL: NLBGS 3 ; 36.1026048 0.0675058411 NL: NLBGS 4 ; 2.86199037 0.00535144399 NL: NLBGS 5 ; 0.0110913874 2.0739042e-05 NL: NLBGS 6 ; 0.000143843779 2.68963842e-07 NL: NLBGS 7 ; 1.00670291e-05 1.8823663e-08 NL: NLBGS 8 ; 2.34205986e-08 4.37926078e-11 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 544.086815 1 NL: NLBGS 2 ; 506.103644 0.930189134 NL: NLBGS 3 ; 37.66376 0.0692238057 NL: NLBGS 4 ; 3.42327333 0.00629177777 NL: NLBGS 5 ; 0.0225600466 4.14640567e-05 NL: NLBGS 6 ; 0.00017040105 3.13187243e-07 NL: NLBGS 7 ; 1.26074273e-05 2.317172e-08 NL: NLBGS 8 ; 1.2239101e-08 2.24947575e-11 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 256.046847 1 NL: NLBGS 2 ; 258.25441 1.00862171 NL: NLBGS 3 ; 16.580322 0.0647550329 NL: NLBGS 4 ; 1.32368588 0.00516970193 NL: NLBGS 5 ; 0.00444365411 1.7354848e-05 NL: NLBGS 6 ; 5.11339102e-05 1.99705291e-07 NL: NLBGS 7 ; 3.67408039e-06 1.43492507e-08 NL: NLBGS 8 ; 8.50899586e-09 3.32321837e-11 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 242.884616 1 NL: NLBGS 2 ; 231.209128 0.951929899 NL: NLBGS 3 ; 18.4819744 0.0760936394 NL: NLBGS 4 ; 1.80708245 0.0074400861 NL: NLBGS 5 ; 0.0103592258 4.2650811e-05 NL: NLBGS 6 ; 0.000168916716 6.95460744e-07 NL: NLBGS 7 ; 1.36439626e-05 5.61746678e-08 NL: NLBGS 8 ; 4.06312665e-08 1.67286291e-10 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 1199.91089 1 NL: NLBGS 2 ; 1210.59169 1.00890133 NL: NLBGS 3 ; 76.8135009 0.0640160044 NL: NLBGS 4 ; 6.00240793 0.00500237808 NL: NLBGS 5 ; 0.0198460018 1.6539563e-05 NL: NLBGS 6 ; 0.000229705135 1.91435161e-07 NL: NLBGS 7 ; 1.61641755e-05 1.34711466e-08 NL: NLBGS 8 ; 3.78466263e-08 3.15411974e-11 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 1135.42777 1 NL: NLBGS 2 ; 1082.45119 0.953342184 NL: NLBGS 3 ; 85.3180484 0.0751417666 NL: NLBGS 4 ; 8.17111519 0.00719650815 NL: NLBGS 5 ; 0.0471581836 4.1533407e-05 NL: NLBGS 6 ; 0.000726707871 6.40030032e-07 NL: NLBGS 7 ; 5.76826634e-05 5.0802583e-08 NL: NLBGS 8 ; 1.74531489e-07 1.53714304e-10 NL: NLBGS 9 ; 6.82561981e-10 6.01149629e-13 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 4596.81178 1 NL: NLBGS 2 ; 4641.85188 1.00979812 NL: NLBGS 3 ; 279.234386 0.0607452294 NL: NLBGS 4 ; 20.1376016 0.00438077575 NL: NLBGS 5 ; 0.0620977592 1.35088757e-05 NL: NLBGS 6 ; 0.000713256539 1.55163312e-07 NL: NLBGS 7 ; 4.65843017e-05 1.01340459e-08 NL: NLBGS 8 ; 1.14411129e-07 2.4889235e-11 NL: NLBGS 9 ; 1.12890979e-09 2.45585384e-13 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 4308.78922 1 NL: NLBGS 2 ; 4117.60132 0.955628394 NL: NLBGS 3 ; 314.553802 0.0730028288 NL: NLBGS 4 ; 27.6516442 0.00641749753 NL: NLBGS 5 ; 0.15997835 3.71283769e-05 NL: NLBGS 6 ; 0.00191439922 4.44300968e-07 NL: NLBGS 7 ; 0.000141273497 3.27872844e-08 NL: NLBGS 8 ; 4.49498065e-07 1.04321201e-10 NL: NLBGS 9 ; 1.58721633e-09 3.68367133e-13 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 1850.75175 1 NL: NLBGS 2 ; 1869.85962 1.01032438 NL: NLBGS 3 ; 108.757532 0.0587639762 NL: NLBGS 4 ; 7.56926922 0.00408983497 NL: NLBGS 5 ; 0.0205768277 1.11180917e-05 NL: NLBGS 6 ; 0.000252720273 1.36550066e-07 NL: NLBGS 7 ; 1.59834641e-05 8.63620103e-09 NL: NLBGS 8 ; 4.30560242e-08 2.32640733e-11 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 1711.81232 1 NL: NLBGS 2 ; 1625.55671 0.949611523 NL: NLBGS 3 ; 133.246795 0.0778396051 NL: NLBGS 4 ; 10.8407644 0.00633291641 NL: NLBGS 5 ; 0.0580616025 3.39182057e-05 NL: NLBGS 6 ; 0.000422830525 2.47007525e-07 NL: NLBGS 7 ; 2.86318498e-05 1.67260449e-08 NL: NLBGS 8 ; 7.76875846e-08 4.53832371e-11 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 590.116582 1 NL: NLBGS 2 ; 596.89267 1.01148263 NL: NLBGS 3 ; 34.4002045 0.0582939126 NL: NLBGS 4 ; 2.3738814 0.00402273292 NL: NLBGS 5 ; 0.00633527682 1.0735636e-05 NL: NLBGS 6 ; 7.72042085e-05 1.30828739e-07 NL: NLBGS 7 ; 4.84775969e-06 8.2149186e-09 NL: NLBGS 8 ; 1.37059963e-08 2.32259128e-11 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 541.871381 1 NL: NLBGS 2 ; 518.324852 0.956545907 NL: NLBGS 3 ; 41.3292465 0.0762713219 NL: NLBGS 4 ; 3.34458795 0.0061722912 NL: NLBGS 5 ; 0.0178849541 3.30059027e-05 NL: NLBGS 6 ; 0.000134842332 2.48845642e-07 NL: NLBGS 7 ; 9.08207474e-06 1.67605728e-08 NL: NLBGS 8 ; 2.61182972e-08 4.82001783e-11 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 527.343524 1 NL: NLBGS 2 ; 533.529294 1.01173006 NL: NLBGS 3 ; 30.6151043 0.0580553338 NL: NLBGS 4 ; 2.09085512 0.00396488253 NL: NLBGS 5 ; 0.00554487896 1.05147379e-05 NL: NLBGS 6 ; 6.81810211e-05 1.29291473e-07 NL: NLBGS 7 ; 4.23555666e-06 8.03187385e-09 NL: NLBGS 8 ; 1.21785713e-08 2.30941896e-11 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 483.576294 1 NL: NLBGS 2 ; 463.501984 0.95848781 NL: NLBGS 3 ; 36.4040597 0.0752809021 NL: NLBGS 4 ; 2.92183708 0.0060421429 NL: NLBGS 5 ; 0.0157103881 3.24879202e-05 NL: NLBGS 6 ; 0.00011698578 2.41917937e-07 NL: NLBGS 7 ; 7.8150022e-06 1.61608464e-08 NL: NLBGS 8 ; 2.28400154e-08 4.7231462e-11 NL: NLBGS Converged ================== AS_point_0.coupled ================== NL: NLBGS 1 ; 316.194523 1 NL: NLBGS 2 ; 320.009443 1.0120651 NL: NLBGS 3 ; 18.1819581 0.0575024449 NL: NLBGS 4 ; 1.23493104 0.00390560542 NL: NLBGS 5 ; 0.00318279267 1.00659323e-05 NL: NLBGS 6 ; 3.92858171e-05 1.24245723e-07 NL: NLBGS 7 ; 2.43074681e-06 7.68750448e-09 NL: NLBGS 8 ; 7.00756213e-09 2.21621869e-11 NL: NLBGS Converged ================== AS_point_1.coupled ================== NL: NLBGS 1 ; 288.84284 1 NL: NLBGS 2 ; 276.826267 0.958397538 NL: NLBGS 3 ; 21.7740483 0.0753837216 NL: NLBGS 4 ; 1.72779277 0.0059817746 NL: NLBGS 5 ; 0.0091697294 3.17464313e-05 NL: NLBGS 6 ; 6.34805872e-05 2.19775526e-07 NL: NLBGS 7 ; 4.18425019e-06 1.4486252e-08 NL: NLBGS 8 ; 1.20817589e-08 4.18281404e-11 NL: NLBGS Converged Optimization terminated successfully (Exit mode 0) Current function value: 0.026596816564823787 Iterations: 17 Function evaluations: 20 Gradient evaluations: 17 Optimization Complete -----------------------------------
# prob.run_model()
print("The fuel burn value is", prob["AS_point_0.fuelburn"][0], "[kg]")
The fuel burn value is 2659.6816564823785 [kg]
print(
"The wingbox mass (excluding the wing_weight_ratio) is",
prob["wing.structural_mass"][0] / surf_dict["wing_weight_ratio"],
"[kg]",
)
The wingbox mass (excluding the wing_weight_ratio) is 1119.5523503688187 [kg]
There is plenty of room for improvement. A finer mesh and a tighter optimization tolerance should be used.